Authentication in laravel
To create registration page in laravel, you’ll need to follow a few steps. Here is basic outline of the process.
Create your project using this command: via the Composer create-project
command:
composer create-project laravel/laravel example-app
Run these commands on the terminal to run your project::
php artisan serve
Create a database file and set the configurations inside .env file in your project:
DB_DATABASE=(Put here your database name) DB_USERNAME=root DB_PASSWORD=password
Laravel follows Model View Controller Structure.
VIEW: The View is responsible for presenting the data to the user in a readable format.
CONTROLLER: The Controller handles the user’s requests and acts as an intermediary between the Model and the View. It receives input from the user, processes it, interacts with the Model to fetch or manipulate data, and then passes the data to the View for rendering.
MODEL: It is responsible for interacting with the database or any other data source, performing data manipulation, and enforcing data validation rules. In Laravel, models are typically created as classes extending the Illuminate\Database\Eloquent\Model
base class. They define the structure of database tables and provide methods to query and manipulate data.
STEP 1: CREATE REGISTER VIEW PAGE FOR REGISTRATION
Here we create a layout file in which we can write common code.
<!DOCTYPE html> <html lang="{{ str_replace('_', '-', app()->getLocale()) }}"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Laravel</title> <!-- Fonts --> <link rel="preconnect" href="https://fonts.bunny.net"> <link href="https://fonts.bunny.net/css?family=figtree:400,600&display=swap" rel="stylesheet" /> <!-- Styles --> </head> <body> <section class="vh-100 gradient-custom"> <div class="container py-5 h-100"> <div class="row justify-content-center align-items-center h-100"> <div class="col-12 col-lg-9 col-xl-7"> <div class="card shadow-2-strong card-registration" style="border-radius: 15px;"> <div class="card-body p-4 p-md-5"> <h3 class="mb-4 pb-2 pb-md-0 mb-md-5">Registration Form</h3> <form method="post" action="register"> {{ csrf_field() }} <div class="row"> <div class="col-md-12 mb-4"> <div class="form-outline"> <input type="text" id="firstName" class="form-control form-control-lg" name="name"> <label class="form-label" for="name">First Name</label> </div> </div> </div> <div class="row"> <div class="col-md-12 mb-4 pb-2"> <div class="form-outline"> <input type="email" id="emailAddress" class="form-control form-control-lg" name="email"> <label class="form-label" for="emailAddress">Email</label> </div> </div> </div> <div class="row"> <div class="col-md-12 mb-4 pb-2"> <div class="form-outline"> <input type="password" id="password" class="form-control form-control-lg" name="password"> <label class="form-label" for="password">Password</label> </div> </div> </div> <div class="mt-4 pt-2"> <input class="btn btn-primary btn-lg" type="submit" value="Submit" /> </div> </form> </div> </div> </div> </div> </div> </section> </body> </html>
STEP 2: CREATE CONTROLLER RELATED TO REGISTER VIEW PAGE:
We need to create a Controller to handle our form data. From View Files, When we submit our form data, it goes to Controller. We can make the controller by using the following command: php artisan make:controller AuthController.
You will find your controller under App > http > Controller > AuthController
namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Register; class AuthController extends Controller { public function register() { return view('registration.register'); } public function store(Request $request) { $credentials = new Register(); $credentials->name = $request->name; $credentials->email = $request->email; $credentials->password= $request->password; $credentials->save(); return redirect()->back(); } }
STEP 3: CREATE ROUTES FOR VIEW AND CONTROLLER FILES:
To access the Registration view files and its related controller we need to create routes in web.php file. Open routes > web.php
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\AuthController; /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider and all of them will | be assigned to the "web" middleware group. Make something great! | */ Route::get('register',[AuthController::class,'register']); Route::post('register',[AuthController::class,'store']);
create the register() to view your page and store() to handle the data. For Example, function store() will handle registration data .In this function, we will store the user’s data and then pass that data to the data table to store it in the database using Model. So we need to create a Model for that particular data table in which we want to store our user’s data.

STEP 4: CREATE MODEL:
We can create a model by using the following command: Php artisan make:model model name
. Import model to your controller.
<?php namespace App\Models; // use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; use Laravel\Sanctum\HasApiTokens; class User extends Authenticatable { use HasApiTokens, HasFactory, Notifiable; /** * The attributes that are mass assignable. * * @var array<int, string> */ protected $table = 'user'; protected $fillable = [ 'name', 'email', 'password', ]; /** * The attributes that should be hidden for serialization. * * @var array<int, string> */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast. * * @var array<string, string> */ protected $casts = [ 'email_verified_at' => 'datetime', 'password' => 'hashed', ]; }
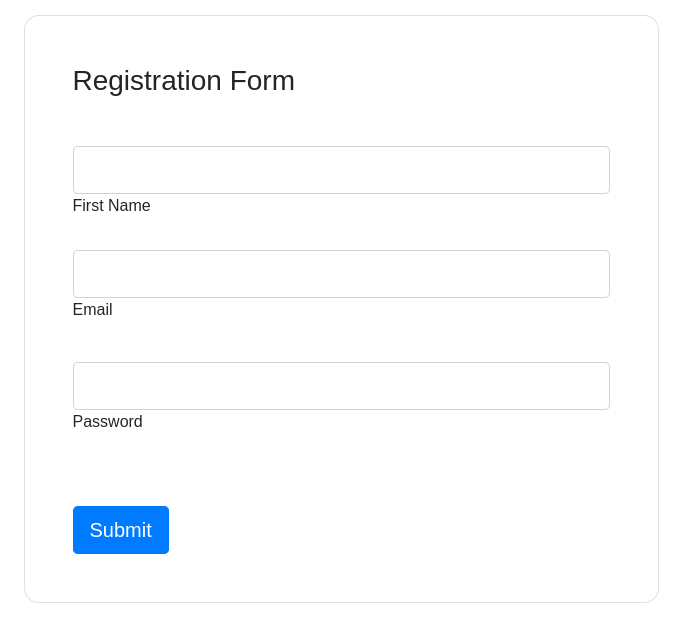
So once the user submits the registration form, the controller action responsible for handling the registration process, it will create a new user record in the database. Then redirect the user to another page, such as a home page or a login page.
LOGIN:
- After successfully done with registration, we can login. Login will also follow the same steps
- If the user login with email id and password, which has been registered, he will be logged in successfully and redirect in home page and in another page.
STEP 1: CREATE LOGIN VIEW PAGE
Here we create a layout file in which we can write common code for login.
<!DOCTYPE html> <html lang="{{ str_replace('_', '-', app()->getLocale()) }}"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Laravel</title> <!-- Fonts --> <link rel="preconnect" href="https://fonts.bunny.net"> <link href="https://fonts.bunny.net/css?family=figtree:400,600&display=swap" rel="stylesheet" /> <!-- Styles --> </head> <body> <section class="vh-100 gradient-custom"> <div class="container py-5 h-100"> <div class="row justify-content-center align-items-center h-100"> <div class="col-12 col-lg-9 col-xl-7"> <div class="card shadow-2-strong card-registration" style="border-radius: 15px;"> <div class="card-body p-4 p-md-5"> <h3 class="mb-4 pb-2 pb-md-0 mb-md-5">Login Form</h3> <div> @if(Session::has('error')) <div class="alert alert-danger" role="alert">{{Session::get('error')}}</div> @endif </div> <form action ="{{ route('login') }}" method="post"> {{ csrf_field() }} <div class="row"> <div class="col-md-12 mb-4 pb-2"> <div class="form-outline"> <input type="email" id="email" class="form-control form-control-lg" name="email"> <label class="form-label" for="emailAddress">Email</label> </div> </div> </div> <div class="row"> <div class="col-md-12 mb-4 pb-2"> <div class="form-outline"> <input type="password" class="form-control form-control-lg" name="password" autocomplete="on"> <label class="form-label" for="password">Password</label> </div> </div> </div> <div class="mt-4 pt-2"> <input class="btn btn-primary btn-lg" type="submit" value="Submit" /> </div> </form> </div> </div> </div> </div> </div> </section> </body> </html>
STEP 2: CREATE CONTROLLER RELATED TO LOGIN VIEW PAGE:
<?php namespace App\Http\Controllers; use Illuminate\Support\Facades\Auth; use Illuminate\Http\Request; use App\Models\Register; use Illuminate\Support\Facades\Hash; class AuthController extends Controller { public function login() { return view('registration.login'); } public function loginPost(Request $request) { $email = $request->email; $password = $request->password; if (Auth::attempt(['email' => $email, 'password' => $password])){ return redirect()->route('home')->with('success', 'login Successful'); }else{ return back()->with('error', 'Incorrect Email and Password'); } } }
STEP 3: CREATE ROUTES FOR VIEW AND CONTROLLER FILES:
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\AuthController; Route::get('login', [AuthController::class,'login'])->name('login'); Route::post('login', [AuthController::class,'loginPost'])->name('login');